- Published on
How to connect to Amazon's Business API
If you need help integrating with e-commerce APIs, get in touch. We spend every day working on tools to speed up and improve e-commerce business processes.
A new Amazon e-commerce API quietly arrived on the scene without much press: Amazon's Business API. The Business API allows Amazon Business customers to streamline and automate operations, including:
- Creating and downloading invoices
- Searching for and evaluating offers for products of interest
- Reconciling business transactions
- Tracking orders and spending
- Managing Business user accounts
The documentation on how to access and use the Business API is limited. We waded through the process so that you don't have to. Here are the steps:
- Create an Amazon Business account if you don't already have one
- Submit a Developer Profile application to get access to the Business API
- Create a Business API application in Developer Central
- Complete the self-authorization flow to get a refresh token
- Make a call to the Business API!
Let's get started.
Create an Amazon Business account
First things first: if you don't have an Amazon Business account, you'll need to create one. Head over to the Amazon Business signup page and follow the on-screen instructions to set up your account.1 It's easy and should only take a few minutes.
Submit your Developer Profile application to get access to the Business API
You'll need to submit a Developer Profile application to get access to the Amazon Business API. To do this, go to the Developer Profile page on Developer Central.
The first part of the application is all business-specific details. Once you've filled that out, take a look at the Data Access section. The first option you will need to select is whether you're a private developer or a public developer. The first dropdown in this section asks whether you're planning to use the Business API to build tools for your own account, or to build tools that you will sell to other companies that use Amazon. If you're not sure which to choose, pick Private developer.
If you previously developed applications with MWS, include your MWS developer ID(s) in the next field. If you built MWS applications that worked in multiple Amazon regions (NA/EU/FE), you will have a separate MWS developer ID for each region. If you never built MWS applications (or don't know what MWS is), this doesn't apply to you.
The next and most important step is choosing which data you want access to, based the roles you request approval for. The Developer Profile includes all the roles for both the Business, Selling Partner, Freight, and Sustainability Certifier APIs. If you only want to develope Business API functionality, the only relevant roles are Business Product Catalog, Business Purchase Reconciliation, and Amazon Business Analytics. See here for a detailed breakdown of the Business API roles.
Complete the rest of the questions, selecting Yes for all of the Yes/No questions2. As long as you didn't select any roles marked "Restricted", the Developer Profile application should be relatively simple. If you did request access to restricted roles, check out this section of our article about accessing to the Selling Partner API for guidance on how to answer the additional PII-related questions in the Developer Profile application.
You can now submit the Developer Profile form. You will receive an email from Amazon when your account is approved. If you didn't request any restricted roles, you will usually get approved within a day.
We've written a lot of developer applications, and Amazon can be pretty picky about the answers to the application questions. If you want help getting access, get in touch and we can work with you to write a successful application.
Business application configuration
Once your developer profile has been approved, you can start the process of creating a Business API app.
AWS IAM setup
You need to do some AWS IAM setup before you can create a Business API application. The IAM setup for the Business API is identical to the IAM setup for the Selling Partner API, so just follow the IAM configuration instructions from our SP API setup post and you should be good to go. Hang on to the AWS access key ID, secret access key, and role ARN you end up with – we'll need them later.
Create a Business API app
Once you're done with IAM configuration, click Add new app client in Developer Central.
Your app config should look something like this, assuming you only want to access the Business API with this application (not the SP API/Freight API/etc):
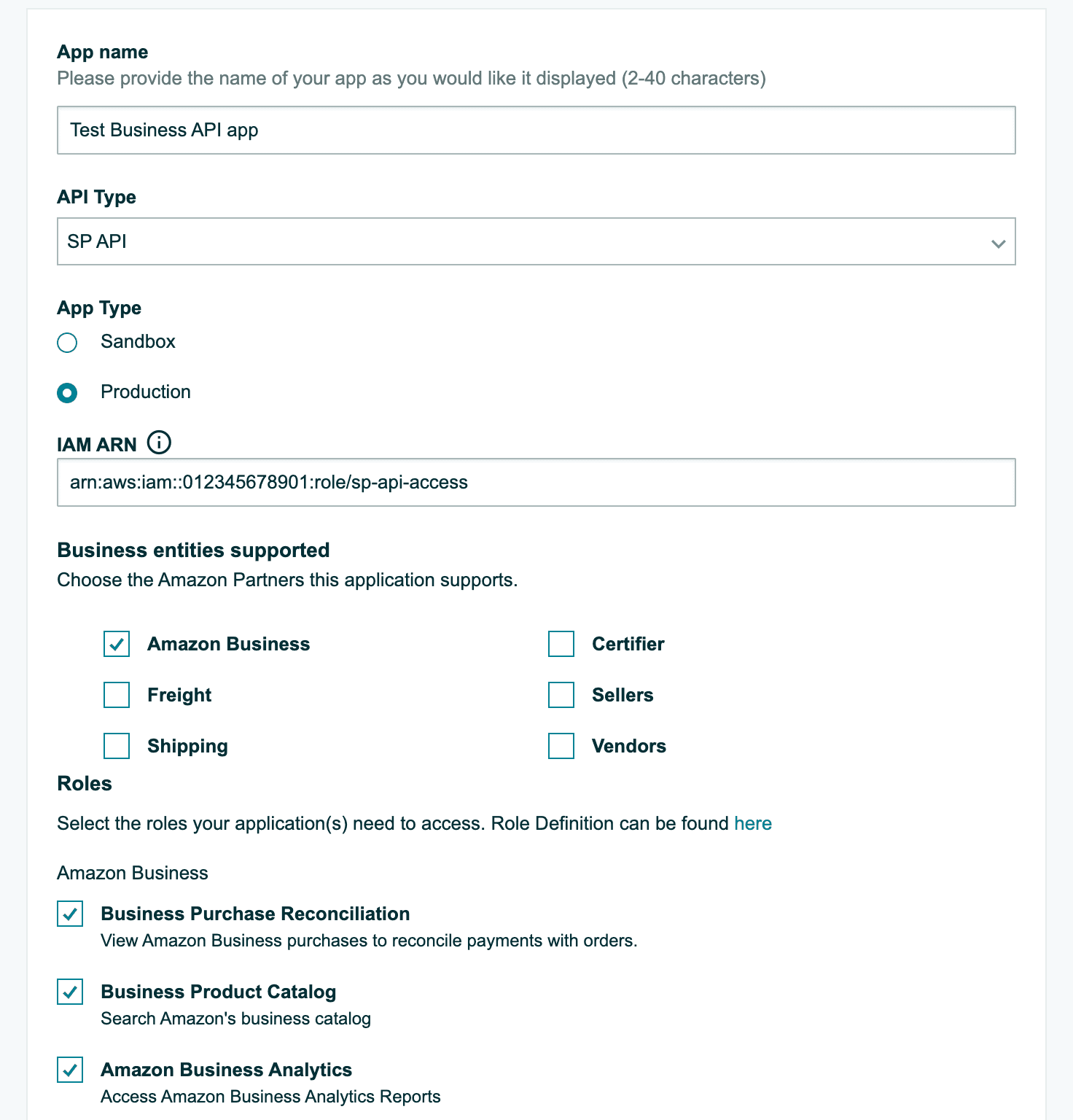
Choose No for the PII delegation question. Unlike Selling Partner API applications, Business API applications all need to have OAuth login/redirect URIs specified. To save you the difficulty of setting those up yourself, we've set up functional login/redirect URLs for you:
- Login URI:
https://www.highsidelabs.co/api/oauth/amazon-business/initiate
- Redirect URI:
https://www.highsidelabs.co/api/oauth/amazon-business/receive
We do not collect any information from these endpoints, and will not retain any of your data. We just set these up to make it easier to get started with the Business API by making the authorization form in the next section work.
You're done! Just click Save and exit and you'll be taken back to the main Developer Central page. You should see your new app listed there. We need to grab a few configuration values for the next step: the app ID, LWA client ID, and LWA client secret. The app ID is right under the app name, on the left. The LWA credentials are hidden inside the View link in the LWA credentials column.

Complete the authorization flow to get a refresh token
To generate a refresh token for your own Business account, use the form below. Enter the app ID, LWA client ID, and LWA client secret from the last step and click Authorize. You will be redirected to the Amazon Business authorization page, and then back to this page once you're approved it. A refresh token will appear in the field below.
Enter the app ID of the Business API application you want to authorize.
If you run into any issues with this step, let us know.
Make a call to the Business API
Once you have a refresh token, you have everything you need to make a request to the Business API! We created a PHP library to make this easier. If you've used our Selling Partner API library, this will be a piece of cake – the interface for our Business API library is nearly identical.
Install the package with composer require highsidelabs/amazon-business-api
.
To make your first Business API call, you'll need some different configuration values:
- The LWA client ID and client secret values from step 3
- The IAM access key ID, secret access key, and role ARN from the AWS setup section
With those values, construct a configuration object:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use AmazonBusinessApi\Configuration;
use AmazonBusinessApi\Endpoint;
$config = new Configuration([
'lwaClientId' => 'amzn1.application-oa2-client....',
'lwaClientSecret' => '8ce1....',
'lwaRefreshToken' => 'Atzr|IwEB....',
'awsAccessKeyId' => 'AKIA....',
'awsSecretAccessKey' => 'OrFW2....',
'roleArn' => 'arn:aws:iam::012345678901:role/business-api-access',
'endpoint' => Endpoint::NA,
]);
Then construct an instance of one of the Business API classes. This example assumes you have access to the Business Product Catalog role.
use AmazonBusinessApi\Api\ProductSearchV20200826Api as ProductSearchApi;
$api = new ProductSearchApi($config);
$res = $api->searchProductsRequest(
product_region: 'US',
locale: 'en_US',
x_amz_user_email: '<your Amazon Business email>',
keywords: ['toothbrush', 'crest'],
);
print_r($res);
Note that we're using named arguments here to make it easier to distinguish which argument is which, since the searchProductsRequest
endpoint has a lot of optional parameters. This is a PHP 8 feature, so if you're using an older version of PHP, you'll need to pass the arguments in the order they appear in the method signature.
Here's the code all together:
<?php
require_once __DIR__ . '/vendor/autoload.php';
use AmazonBusinessApi\Api\ProductSearchV20200826Api as ProductSearchApi;
use AmazonBusinessApi\Configuration;
use AmazonBusinessApi\Endpoint;
$config = new Configuration([
'lwaClientId' => 'amzn1.application-oa2-client....',
'lwaClientSecret' => '8ce1....',
'lwaRefreshToken' => 'Atzr|IwEB....',
'awsAccessKeyId' => 'AKIA....',
'awsSecretAccessKey' => 'OrFW2....',
'roleArn' => 'arn:aws:iam::012345678901:role/business-api-access',
'endpoint' => Endpoint::NA,
]);
$api = new ProductSearchApi($config);
$res = $api->searchProductsRequest(
product_region: 'US',
locale: 'en_US',
x_amz_user_email: '<your Amazon Business email>',
keywords: ['toothbrush', 'crest'],
);
print_r($res->products[0]);
And that's it! To get the first matching product, you can access the products
array:
$res->products[0];
There is comprehensive documentation available from us for the library, and from Amazon for the Business API as a whole.
If you need help with any of the steps in this guide, shoot us an email at hi@highsidelabs.co. We specialize in developing custom e-commerce integrations, and can get you back on track quickly. If you want to get notified next time we publish a blog post, subscribe to our newsletter!
Footnotes
If you enter the email for your current (non-Business) Amazon account, you will be asked if you want to convert your account to a Business account. ↩
Disclaimer: I am not a lawyer. None of this is legal advice, and you should answer all the questions in your Developer application truthfully, or risk breaking Amazon's terms of service. ↩